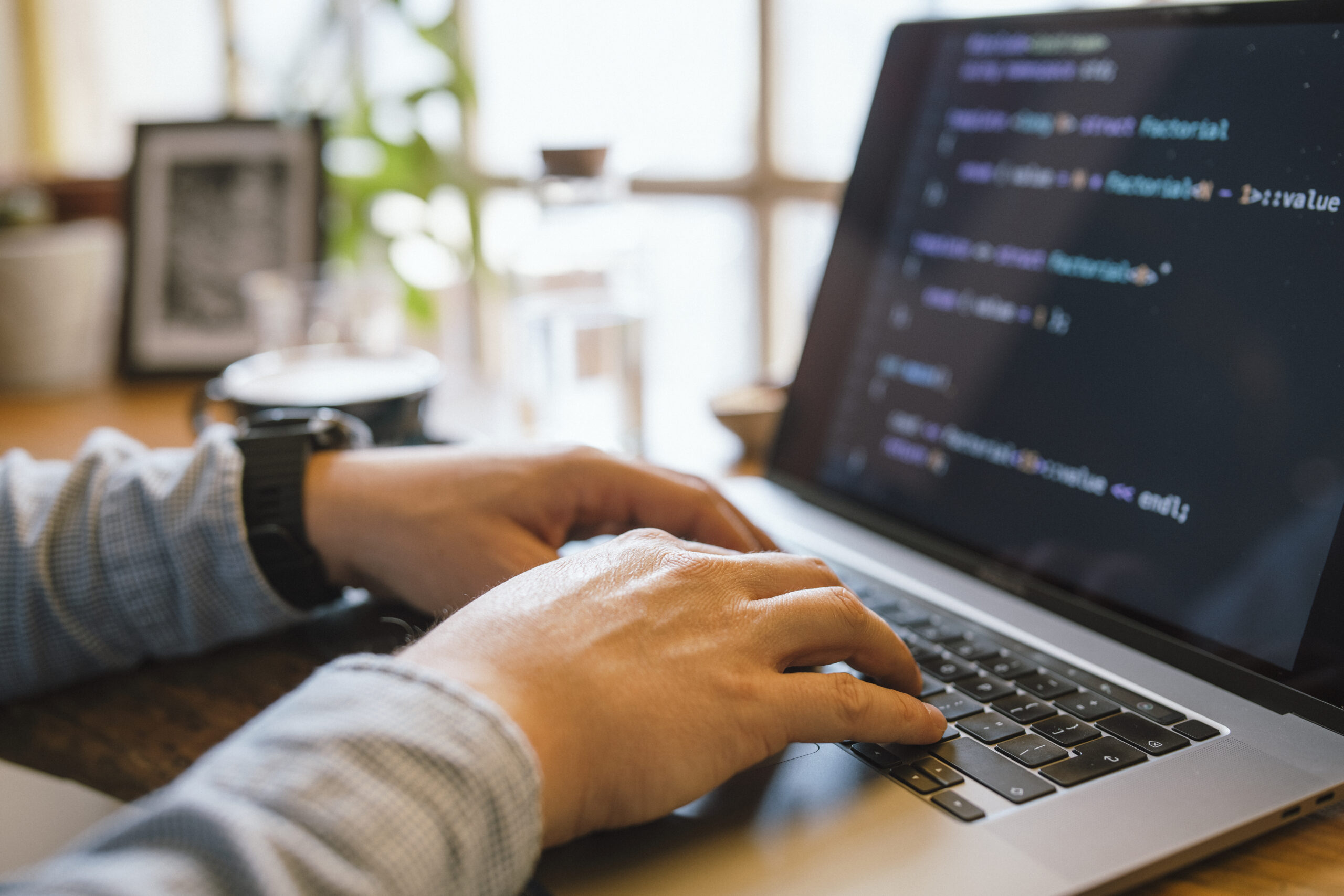
Debugging is One of the more crucial — still often ignored — expertise in the developer’s toolkit. It's actually not just about correcting damaged code; it’s about comprehending how and why issues go Improper, and Finding out to Consider methodically to resolve troubles proficiently. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While writing code is a person Component of growth, realizing how to connect with it effectively all through execution is Similarly essential. Modern progress environments arrive equipped with effective debugging capabilities — but quite a few developers only scratch the area of what these equipment can do.
Choose, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments help you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and also modify code on the fly. When applied effectively, they Allow you to notice precisely how your code behaves through execution, which can be a must have for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, perspective actual-time general performance metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can switch frustrating UI concerns into workable responsibilities.
For backend or method-stage builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging procedures and memory management. Mastering these tools might have a steeper Finding out curve but pays off when debugging general performance issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into comfy with Edition Management devices like Git to be familiar with code history, discover the exact instant bugs were being released, and isolate problematic changes.
In the end, mastering your resources implies heading further than default configurations and shortcuts — it’s about developing an personal understanding of your advancement setting to ensure that when concerns come up, you’re not misplaced at nighttime. The higher you understand your resources, the greater time you may shell out resolving the particular dilemma as an alternative to fumbling by way of the procedure.
Reproduce the condition
One of the more significant — and sometimes neglected — measures in successful debugging is reproducing the situation. Ahead of jumping into the code or making guesses, builders need to have to make a regular surroundings or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a game of probability, typically resulting in wasted time and fragile code improvements.
Step one in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What actions led to The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it will become to isolate the exact ailments below which the bug takes place.
After you’ve gathered adequate details, seek to recreate the challenge in your neighborhood setting. This may suggest inputting a similar details, simulating equivalent person interactions, or mimicking method states. If The problem seems intermittently, contemplate crafting automatic checks that replicate the edge situations or point out transitions concerned. These assessments don't just assist expose the problem but in addition protect against regressions in the future.
Often, The difficulty might be setting-particular — it would transpire only on certain operating programs, browsers, or underneath particular configurations. Utilizing applications like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be currently halfway to fixing it. Having a reproducible situation, You need to use your debugging equipment far more successfully, test possible fixes safely, and communicate much more clearly together with your team or end users. It turns an summary grievance into a concrete challenge — Which’s where by builders thrive.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most respected clues a developer has when some thing goes wrong. Rather than seeing them as frustrating interruptions, builders really should understand to treat error messages as immediate communications through the process. They typically let you know precisely what transpired, wherever it occurred, and occasionally even why it transpired — if you know the way to interpret them.
Start out by reading through the message diligently and in complete. Lots of developers, particularly when under time force, glance at the main line and quickly commence making assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into engines like google — study and realize them first.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it position to a specific file and line variety? What module or function induced it? These thoughts can guidebook your investigation and issue you towards the responsible code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable patterns, and learning to recognize these can considerably speed up your debugging method.
Some glitches are vague or generic, and in People instances, it’s critical to look at the context by which the error transpired. Look at related log entries, input values, and recent changes within the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger concerns and supply hints about probable bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a much more effective and assured developer.
Use Logging Properly
Logging is The most strong instruments in a very developer’s debugging toolkit. When made use of efficiently, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s happening under the hood without needing to pause execution or step throughout the code line by line.
An excellent logging method begins with realizing what to log and at what level. Typical logging levels include DEBUG, Facts, WARN, Developers blog Mistake, and Deadly. Use DEBUG for thorough diagnostic details in the course of advancement, INFO for general situations (like successful get started-ups), Alert for likely concerns that don’t break the applying, ERROR for actual problems, and FATAL in the event the technique can’t proceed.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your process. Target important situations, condition modifications, enter/output values, and demanding decision factors inside your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you monitor how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without halting the program. They’re Specifically important in manufacturing environments where by stepping by means of code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, smart logging is about equilibrium and clarity. Using a very well-thought-out logging strategy, you could reduce the time it requires to identify issues, get further visibility into your applications, and Enhance the Over-all maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a specialized undertaking—it is a form of investigation. To successfully discover and deal with bugs, builders must method the method similar to a detective resolving a secret. This state of mind aids break down intricate difficulties into workable pieces and follow clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the condition: mistake messages, incorrect output, or performance issues. Just like a detective surveys a criminal offense scene, acquire as much pertinent data as it is possible to with no jumping to conclusions. Use logs, examination circumstances, and user studies to piece collectively a clear picture of what’s happening.
Next, variety hypotheses. Talk to you: What may very well be resulting in this actions? Have any variations not long ago been designed on the codebase? Has this concern occurred before less than related conditions? The objective is always to narrow down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble in a managed setting. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you nearer to the truth.
Pay near interest to compact information. Bugs frequently disguise while in the minimum predicted places—just like a missing semicolon, an off-by-just one error, or simply a race issue. Be thorough and individual, resisting the urge to patch The difficulty with no completely being familiar with it. Short term fixes may perhaps conceal the actual issue, just for it to resurface later.
And finally, keep notes on Whatever you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential challenges and assist Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden concerns in advanced units.
Create Exams
Producing tests is one of the most effective strategies to help your debugging skills and General growth effectiveness. Assessments don't just help catch bugs early and also function a security Web that offers you assurance when making modifications for your codebase. A effectively-examined application is easier to debug as it means that you can pinpoint particularly where by and when a dilemma takes place.
Get started with device assessments, which target specific features or modules. These modest, isolated checks can immediately expose no matter if a certain piece of logic is Functioning as anticipated. When a test fails, you immediately know where by to glimpse, significantly reducing some time expended debugging. Unit exams are especially useful for catching regression bugs—challenges that reappear immediately after Earlier getting set.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable make certain that numerous aspects of your software function together efficiently. They’re specially beneficial for catching bugs that occur in advanced techniques with numerous factors or providers interacting. If something breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what circumstances.
Crafting exams also forces you to definitely Consider critically regarding your code. To test a aspect appropriately, you need to grasp its inputs, expected outputs, and edge situations. This level of understanding Obviously leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the test fails persistently, you'll be able to center on fixing the bug and observe your take a look at pass when the issue is solved. This solution ensures that the identical bug doesn’t return Down the road.
In short, creating checks turns debugging from the irritating guessing match right into a structured and predictable system—assisting you catch far more bugs, speedier and more reliably.
Consider Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the issue—watching your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping away. Using breaks will help you reset your brain, cut down frustration, and often see The difficulty from the new standpoint.
If you're much too near the code for far too very long, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code that you wrote just several hours before. In this particular condition, your brain gets to be much less efficient at problem-resolving. A brief walk, a coffee crack, or maybe switching to another undertaking for ten–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work from the qualifications.
Breaks also enable avert burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You would possibly abruptly see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten moment break. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily below limited deadlines, however it essentially leads to speedier and more effective debugging Over time.
In brief, getting breaks is not a sign of weak point—it’s a sensible method. It offers your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, every one can instruct you something beneficial in case you make the effort to replicate and review what went wrong.
Begin by asking oneself a number of critical thoughts as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it have been caught previously with greater procedures like unit screening, code evaluations, or logging? The solutions usually reveal blind spots inside your workflow or comprehending and assist you to Develop stronger coding routines moving ahead.
Documenting bugs will also be a great habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see styles—recurring troubles or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've realized from a bug along with your peers is often Specially effective. Whether or not it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact situation boosts team performance and cultivates a more powerful Discovering society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. In fact, several of the best developers are not the ones who generate excellent code, but individuals that continually master from their blunders.
In the end, Every single bug you fix adds a different layer for your ability established. So subsequent time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.